The Swagger UI¶
If you installed connexion using the swagger-ui
extra, a Swagger UI is available for each
API, providing interactive documentation. By default the UI is hosted at {base_path}/ui/
where base_path`
is the base path of the API.
https://{host}/{base_path}/ui/
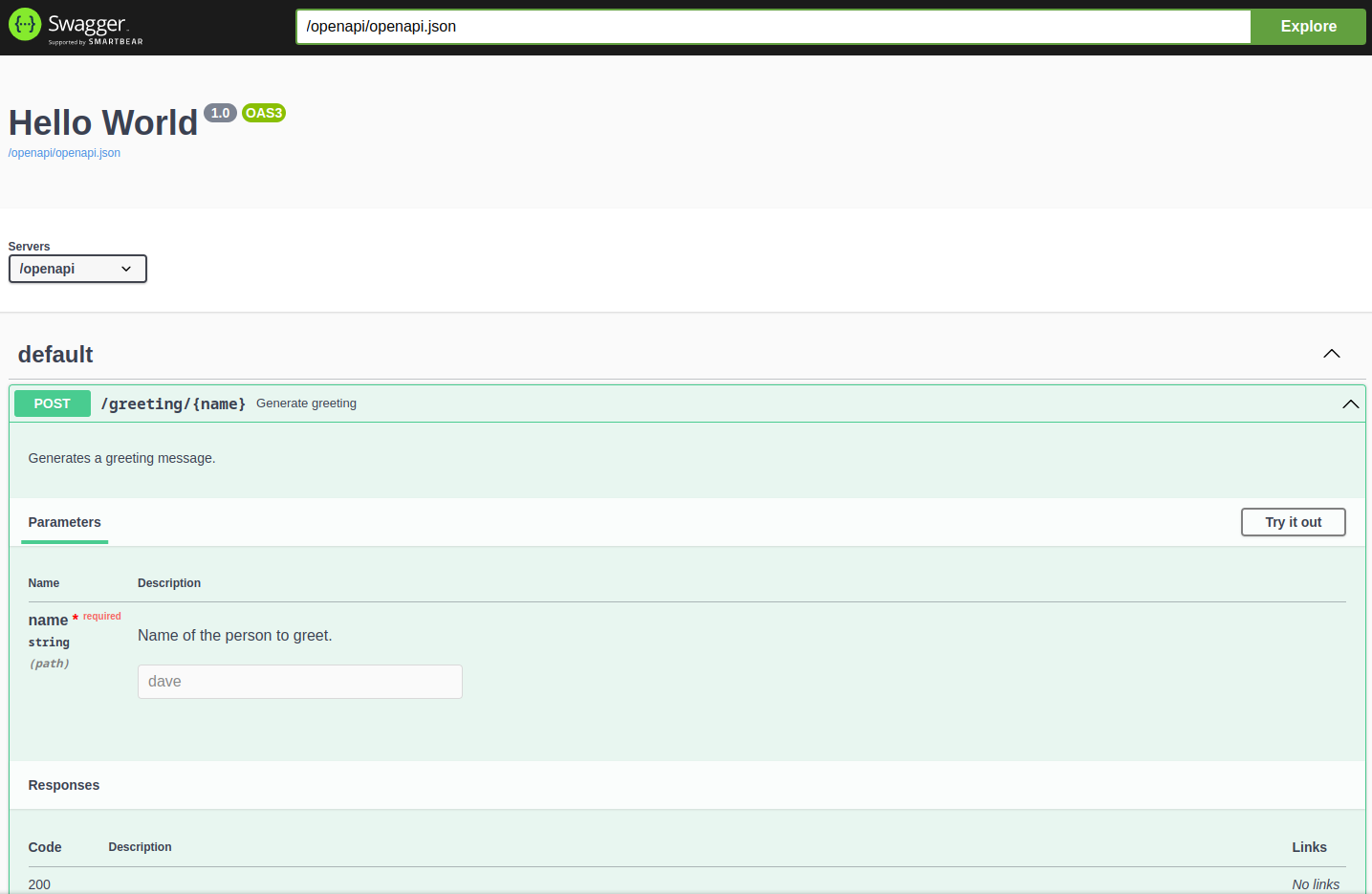
Configuring the Swagger UI¶
You can change this path through the swagger_ui_options
argument, either whe instantiating
your application, or when adding your api:
from connexion import AsyncApp
from connexion.options import SwaggerUIOptions
options = SwaggerUIOptions(swagger_ui_path="/docs")
app = AsyncApp(__name__, swagger_ui_options=options)
app.add_api("openapi.yaml", swagger_ui_options=options)
from connexion import FlaskApp
from connexion.options import SwaggerUIOptions
options = SwaggerUIOptions(swagger_ui_path="/docs")
app = FlaskApp(__name__, swagger_ui_options=options)
app.add_api("openapi.yaml", swagger_ui_options=options)
from asgi_framework import App
from connexion import ConnexionMiddleware
from connexion.options import SwaggerUIOptions
options = SwaggerUIOptions(swagger_ui_path="/docs")
app = App(__name__)
app = ConnexionMiddleware(app, swagger_ui_options=options)
app.add_api("openapi.yaml", swagger_ui_options=options):
For a description of all available options, check the SwaggerUIOptions
class.
View a detailed reference of the SwaggerUIOptions
class
- class connexion.options.SwaggerUIOptions(serve_spec: bool = True, spec_path: ~typing.Optional[str] = None, swagger_ui: bool = True, swagger_ui_config: dict = <factory>, swagger_ui_path: str = '/ui', swagger_ui_template_dir: ~typing.Optional[str] = None, swagger_ui_template_arguments: dict = <factory>)¶
Options to configure the Swagger UI.
- Parameters:
serve_spec – Whether to serve the Swagger / OpenAPI Specification
spec_path – Where to serve the Swagger / OpenAPI Specification
swagger_ui – Whether to serve the Swagger UI
swagger_ui_path – Where to serve the Swagger UI
swagger_ui_config – Options to configure the Swagger UI. See https://swagger.io/docs/open-source-tools/swagger-ui/usage/configuration for an overview of the available options.
swagger_ui_template_dir – Directory with static files to use to serve Swagger UI
swagger_ui_template_arguments – Arguments passed to the Swagger UI template. Useful when providing your own template dir with additional template arguments.